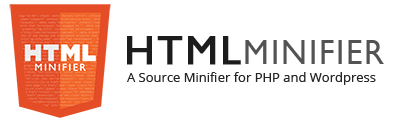
Description
HTML Minifier is a server-side source code minifier, reorganiser and caching tool and that is available both as a PHP class and as a WordPress plugin. It is designed to optimise HTML, CSS and Javascript output sent out to the client by removing whitespace, and by reorganising and / or merging <link>
, <style>
and <script>
tags scattered across HTML pages that are built dynamically on server-side applications.
A variety of optimisation options and minification styles are available in the plugin, and they can be selected from or toggled depending on the user’s needs. To see the full list of options, scroll down to the Minification Options section.
Feel free to use the code any way you like. It is distributed under the GNU Lesser General Public License v3.0.
Usage Instructions These instructions are for the PHP version. To learn more about the WordPress version, install the plugin and go to Settings > HTML Minifier.
HTMLMinifier.php is compatible with all platforms that run PHP on the server backend. To use it, download the file and include the file in your code. Then, call HTMLMinifier::process()
and pass it the HTML source that you want to compress.
<?php
require_once 'mycodefolder/HTMLMinifier.php';
$html = file_get_contents('myhtmlpage.html'); // HTML source to be minified.
echo HTMLMinifier::process($html); // Minified version of the page.
?>
By default, HTMLMinifier::process()
will only apply the safest minification options (for more details, refer to Minification Options). If the appropriate settings are toggled, it can also remove extra whitespaces and comments inside <style>
and <script>
tags. If you want to modify the default compression options, pass an associative array to the function as the second argument.
echo HTMLMinifier::process(
$html,
array(
'shift_script_tags_to_bottom' => true,
'compression_ignore_script_tags' => false,
'compression_mode' => 'all_whitespace'
)
);
If you are using v3.0.0 and above, certain option settings are nested inside others, as they are dependent on a parent setting. For example, combine_javascript_in_script_tags only works if shift_script_tags_to_bottom is enabled, so you will have to nest it into an array and set it to shift_script_tags_to_bottom (see example below). This will cause both attributes to be enabled. For a list of attributes that have dependencies, refer to Minification Options.
echo HTMLMinifier::process(
$html,
array(
'shift_script_tags_to_bottom' => array( 'combine_javascript_in_script_tags' => true );
'compression_ignore_script_tags' => false,
'compression_mode' => 'all_whitespace'
)
);
As of v2.0.0, you can also pass a third argument $cache_key to the HTMLMinifier::process()
. If $CacheFolder
(see below for more details) is properly set up, this will automatically cache the result of HTMLMinifier::process()
under $cache_key. Depending on how your site is set-up, however, it might be more effective to use the HTMLMinifier::cache()
function directly instead. For more details, see Caching Options.
echo HTMLMinifier::process(
$html,
array(
'shift_script_tags_to_bottom' => true,
'compression_ignore_script_tags' => false,
'compression_mode' => 'all_whitespace'
),
'/web/html-minifier/' // The cached page will be saved under this key.
);
Minification Options
Below is a list of all the different options you can pass to HTMLMinifier::process()
as the second argument:
General Options | |
---|---|
Option & Description | Default Value |
clean_html_comments Removes all HTML comments, except those that contain conditional comments. | true |
merge_multiple_head_tags If there are multiple | true |
merge_multiple_body_tags If there are multiple | true |
show_signature Adds a comment at the end of the minified output saying the source was minified by HTML Minifier. | true |
compression_mode How whitespace in the source is compressed. Takes 3 possible string values:
| all_whitespace_not_newlines |
On-page CSS Options | |
---|---|
Option & Description | Default Value |
clean_css_comments Removes all comments inside | true |
remove_comments_with_cdata_tags_css version 3 only In XHTML, content inside | false |
shift_link_tags_to_head Convenience option for pages that are built dynamically in the backend. Moves all | true |
ignore_link_schema_tags version 3 only
| true |
shift_meta_tags_to_head version 3 only Convenience option for pages that are built dynamically in the backend. Moves any | true |
ignore_meta_schema_tags version 3 only
| true |
shift_style_tags_to_head Convenience option for pages that are built dynamically in the backend. Moves any | true |
combine_style_tags Combines all of the on-page CSS inside separate | false |
On-page Javascript Options | |
---|---|
Option & Description | Default Value |
clean_js_comments Removes all comments inside | true |
remove_comments_with_cdata_tags_js version 3 only In XHTML, content inside | false |
compression_ignore_script_tags deprecated as of 3.0.8 Will not apply the active compression_mode to content within Deprecated since Version 3.0.8. Use compression_ignored_tags. | true |
compression_ignored_tags version 3.0.8 and above Array containing a list of all the tags (in lowercase) to skip compression for. This is to prevent HTML Minifier from messing with the behaviour of tags like <textarea> and <pre>, where removed whitespace can affect displayed content. This replaces the functionality of compression_ignore_javascript_tags, since you can also ignore <script> tags with this option. | [ textarea, pre, script ] |
shift_script_tags_to_bottom Convenience option for pages that are built dynamically in the backend. Moves all | false |
combine_javascript_in_script_tags Combines all of the on-page Javascript inside separate | false |
ignore_async_and_defer_tags version 3 only
| false |
Caching Options
Since Version 2.0.0, the PHP version of HTML Minifier includes a caching function. Before this function is usable, however, you need to set the static HTMLMinifier::$CacheFolder
property somewhere in your PHP script. The path points to the folder that you want to save your cached HTML files in.
require_once 'mycodefolder/HTMLMinifier.php';
HTMLMinifier::$CacheFolder = __DIR__; // Cached files are stored in 'mycodefolder'.
Once HTMLMinifier::$CacheFolder
is set, the HTMLMinifier::cache()
function will become usable. The function allows a user to store and retrieve cached results, and is designed to cache dynamically-generated HTML pages.
mixed HTMLMinifier::cache( string $key [, mixed $arg = null ] )
The function works differently depending on whether the optional $arg parameter is passed. If it is not, the function returns a cached HTML file identified by the $key parameter. If the second string argument is passed, the function saves the file into path set in HTMLMinifier::$CacheFolder
(you have to set this yourself!) and returns the length of the string.
The expiry time of cached HTML files is set in $CacheExpiry
in seconds. By default, it is set to 86400 (one day). If a file is older than $CacheExpiry
, a new page will be generated and saved even if a cached file exists.
Caching a File
// Caches output and returns size of $yourHtmlFileData.
HTMLMinifier::cache('/web/html-minifier/', $yourHtmlFileData);
Retrieving a Cached File (If Any)
HTMLMinifier::cache('/web/html-minifier/'); // Returns the data that was cached.
Minifying CSS / JS Resources
If you have a source that contains only CSS or Javascript, you have to use HTMLMinifier::minify_rsc()
to minify it (HTMLMinifier::process()
only processes CSS and Javascript when they are inside the respective tags).
string HTMLMinifier::minify_rsc( string $source, string $file_ext [, array $options = HTMLMinifier::$Defaults, string $cache_key ] )
$source
is the source string, and $file_ext
is a string containing the file extension of the source (i.e. 'css' or 'js'). $ext
is an array containing the options in Minification Options, and if $cache_key
is provided, the minified file will be cached by HTML Minifier (provided file caching is properly set up).
Update Instructions
If you are using the PHP version of HTML Minifier and are updating from v2 to v3, do take note that some settings have been nested inside others, so please update your configurations accordingly.
WordPress Plugin
The WordPress plugin version of HTML Minifier is inside html-minifier.zip. To use it, download the file, unzip it inside your WordPress plugin folder (which is wp-content/plugins by default), and activate the plugin inside wp-admin.
Minification options in the WordPress plugin are available under Settings › HTML Minifier in wp-admin once the plugin is activated.
Feedback & Bug Report
Any and all comments (especially about bugs) will be appreciated. Please send all your feedback or bug reports to our contact page. Alternatively, you can contribute to the project through GitHub.